Application Secure Software Development
Introduction
This procedure guide is deprecated. It was written for the 3.7 version of VT’s Minimum Security Standard.
It is the job of application developers to design secure code in their projects. However, not all web developers are taught how to accomplish this. In order to secure an application, one must run security tests to find problem areas in the application code. Tests can be run through the use of static code scanners, which automate the security testing process for the developers.
Additionally, it’s good to know the best practices for secure web development. From input sanitization to proper security configurations, these skills reduce and prevent the number of coding errors. Fewer errors means fewer points of entry for cyberattacks.
Virginia Tech Security Standards
The following standards apply to all medium and high risk applications.
Standard 1: Secure Design
1.1: Application code will be designed with security in mind, reducing and preventing security vulnerabilities.
For more information and guidance regarding web development security practices, please see the knowledge base (KB) article, Implementing Web Development Site Security.
Standard 2: Code Reviews
2.1: All application code is to be reviewed for security vulnerabilities before deployment.
The use of code analysis tools is recommended for this purpose.
2.2: All application vulnerabilities are to be resolved before deployment.
Using Static Code Analysis Tools
Code analysis tools enable developers to check their code for common security vulnerabilities. Virginia Tech web app developers are recommended to use static code-checking tools, but they can also request a security review by the IT Security Office. To learn more about the IT Security Office’s security reviews, please see the Application Security Reviews documentation.
Static code analysis tools analyze an application’s source code, providing immediate feedback to developers. This is often used before deployment to detect issues and vulnerabilities. There are many free and commercial options. Below are some free, open-source options and their supported coding languages.
- VisualCodeGrepper: C, C++, C#, VB, PHP, Java, PL/SQL, and COBOL.
- Brakeman: Ruby.
- Flawfinder: C and C++.
- Bandit: Python.
OWASP
OWASP stands for the Open-source Web Application Security Project. It is a non-profit organization that creates open-source security-focused projects that are by the community, for the community. They focus on web application development, providing relevant tools, resources, educational materials, training, and community networking.
All of OWASP’s projects are created and maintained by volunteers, and they are frequently updated to keep up with current trends and relevant cyber security threats.
OWASP Top Ten
The OWASP Top Ten is a document of the top ten most critical security vulnerabilities for web applications. It serves as a great resource for keeping up with current security trends as well as getting started with web app security.
Zed Attack Proxy
The OWASP Zed Attack Proxy (ZAP) is a free, open-source web application scanner. Its website contains the application download, a quick start guide, video tutorials, documentation, and more. The OWASP community has also created add-ons that can be used to expand ZAP’s usage.
Secure Coding Practices Guide
The OWASP Secure Coding Practices Quick Reference Guide is a document that lists secure development practices that are applicable to all coding projects, regardless of programming language. This concise, easy-to-read document is only 17 pages long and is perfect for developers of all skill levels.
Security Knowledge Framework
The OWASP Security Knowledge Framework is a Python-Flask/Angular web application that serves as a training tool for developers. It can be run in a Kubernetes cluster or provided as a Software-as-a-Service (SAAS).
- Integrates OWASP’s Mobile and Web Application Verification Standards.
- Contains over 150 interactive labs.
- Includes code examples.
- Comes with a vast knowledge base of vulnerability information, including best management strategies.
Web Security Testing Guide
The OWASP Web Security Testing Guide is a cyber security resource for web developers and security professionals. It goes through the best web application testing practices used by security professionals worldwide.
Specific Secure Coding Guidelines
PortSwigger Web Security Academy
The PortSwigger Web Security Academy is a free resource for anyone that wishes to know more about cyber security. Their articles teach in-depth about common attacks and mitigation strategies.
- Interactive labs.
- Code examples.
- In-depth topic exploration.
Articles in the Web Security Academy include:
- Finding and exploiting blind XXE vulnerabilities
- How to prevent XSS
- How to prevent OAuth authentication vulnerabilities
- How to identify and exploit HTTP host header vulnerabilities
- Defending against CSRF with SameSite cookies
Secure Coding Courses
To train in secure coding practices, there are courses that offer certifications:
Carnegie Mellon University CERT
Video Resources
For further information on secure coding from industry specialists, you may watch some of the following recordings from past developer conferences and events. Each video has the length next to the title in parentheses.
Best Practices
These videos cover the best practices of industry professionals. They provide an in-depth discussion of web application security without specifically targeting a programming language.
Day of Shecurity Secure Coding Best Practices (23:09)
RSA Conference Coding Secure Code (45:20)
Carnegie Mellon University Secure Coding Best Practices (31:13)
CppCon 2018: Secure Coding Best Practices Part 1 (59:55)
CppCon 2018: Secure Coding Best Practices Part 2 (1:02:30)
Specialized Topics
These videos provide specialized security advice for different web setups or vulnerabilities. Videos include code examples and tool walkthroughs.
Securing WordPress Sites (14:01)
- iThemes Security plugin
- SSL certificates
Web App Security: 10 Developer Topics (56:25)
- Secret Management
- SQL Injection Mitigation
- Content-type Checking
- XXE Attack Mitigation
- HTTP Tampering Mitigation
- JWT Token Management
- Cross-Origin Resource Sharing (CORS) Management
- Password Security and Hashing
Writing Secure JavaScript (24:15)
SQL Injection Vulnerability Web Security Academy Lab #2 Walkthrough (7:00)
Fixing a Common XSS Vulnerability (4:56)
Cryptography Concepts for Developers (11:54)
- Hashing and Salting
- HMAC
- Symmetric and Asymmetric Encryption
Procedures
Conducting Static Code Analysis
- Install a static code analysis tool of your choice. Below are some free, open-source options.
- VisualCodeGrepper: C, C++, C#, VB, PHP, Java, PL/SQL, and COBOL.
- Brakeman: Ruby.
- Flawfinder: C and C++.
- Bandit: Python.
- Run the tool. Consult your tool’s documentation for usage instructions.
- Make changes to fix issues that the tool has found before deployment.
- Run the tool again to ensure the issues have been solved.
Installing Zed Attack Proxy (ZAP)
- Download and run the appropriate installer from the Download page.
- Once the installation is complete, launch ZAP and read the license terms. Click Agree to accept the terms. ZAP should run automatically once installation is finished.
- If you’re installing on macOS, the installation will be blocked by Apple because OWASP ZAP is not currently a verified developer. To circumvent this warning, follow these additional steps.
- Go to System Preferences > Security & Privacy. You will see a message saying that “OWASP ZAP” was blocked.
- Next to the message, click Open anyway to trust the installer.
- ZAP will ask if you want to persist the ZAP session. By default, ZAP sessions are always recorded to disk in a HSQLDB database with a default name and location. If you do not persist the session, those files are deleted when you exit ZAP.
If you choose to persist a session, the session information will be saved in the local database so you can access it later, and you will be able to provide custom names and locations for saving the files.
If you do not know which to pick at the moment, then select No, I do not want to persist this session at this moment in time and click Start in the bottom left.
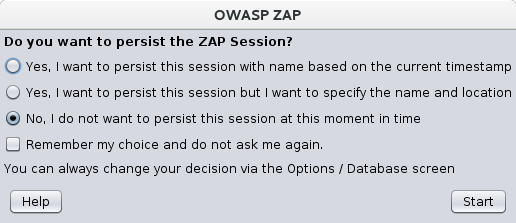
Running an Automated Scan in ZAP
Automated scans are managed in the Quick Start tab. Please remember to only run scans on applications you have permission to test.
- Start ZAP and click the Quick Start tab of the workspace window.
- Click Automated Scan.
- In the URL to attack text box, enter the full URL of the web application you want to attack.
- Click Attack.
Scan results can be viewed in different sections of ZAP.
- To examine a tree view of the explored pages, click the Sites tab in the tree window. You can expand the nodes to see the individual URLs accessed.
- Possible issues and vulnerabilities can be found in the Alerts tab.
Resources
OWASP Security Knowledge Framework
OWASP Web Security Testing Guide